IoT Design with Cypress PSoC® 6 MCUs and Wi-Fi/Bluetooth using Arm® Mbed™
#
Lesson
GitHub Project
0
Introduction
1
Developer Resources
2
Your First Project & The Blinking Thread
https://github.com/iotexpert/mouser-mbed-02.git
3
Display Thread
https://github.com/iotexpert/mouser-mbed-03.git
4
Temperature Thread
https://github.com/iotexpert/mouser-mbed-04.git
5
CapSense Thread
https://github.com/iotexpert/mouser-mbed-05.git
6
WiFi & NTP Thread
https://github.com/iotexpert/mouser-mbed-06.git
7
The CY8CKIT-062-WiFi-BT
https://github.com/iotexpert/mouser-mbed-07.git
8
Amazon AWS MQTT Thread - Part1
https://github.com/iotexpert/mouser-mbed-08.git
9
Amazon AWS MQTT Thread - Part2
https://github.com/iotexpert/mouser-mbed-09.git
You can “mbed import https://github.com/iotexpert/mouser-mbed-09.git“ to make a copy of the project in your workspace.
The final architecture of the thermostat looks like this.
Summary
In this lesson we will plumb in the ability to talk to the Amazon Web Services MQTT broker. Specifically to publish messages from the broker to change the setPoint of the thermostat.
To implement this I will:
- Discuss MQTT
- Import Lesson 07
- Add the Cypress AWS IoT Middleware
- Add the Cypress Connectivity Utilities Library
- Create an AWS Policy, Thing, and Certificate
- Modify the keys to “C” format
- Create and Code awsThread.h
- Create and Code awsThread.cpp
- Update main.cpp
- Build, Program and Test
MQTT
There are five basic ideas that you need to understand when using MQTT.
- (Message) Broker
- Topic
- Subscriber
- Publisher
- Messages
The Broker is a TCP/IP server sitting at the middle of an MQTT network. It is responsible for receiving Messages from Publishers and forwarding them on to Subscribers. A broker can run multiple simultaneous communication channels called Topics.
Topics are ad-hoc (application semantics) and are just a string of characters … like “setPoint” or “thing/setPoint”
Messages are just a string of bytes. Typically formatted as JSON. This is by convention and is not a requirement.
MQTT clients can be Publishers or Subscribers or both.
Import Lesson 07
Start by importing Lesson 07.
https://github.com/iotexpert/mouser-mbed-07.git
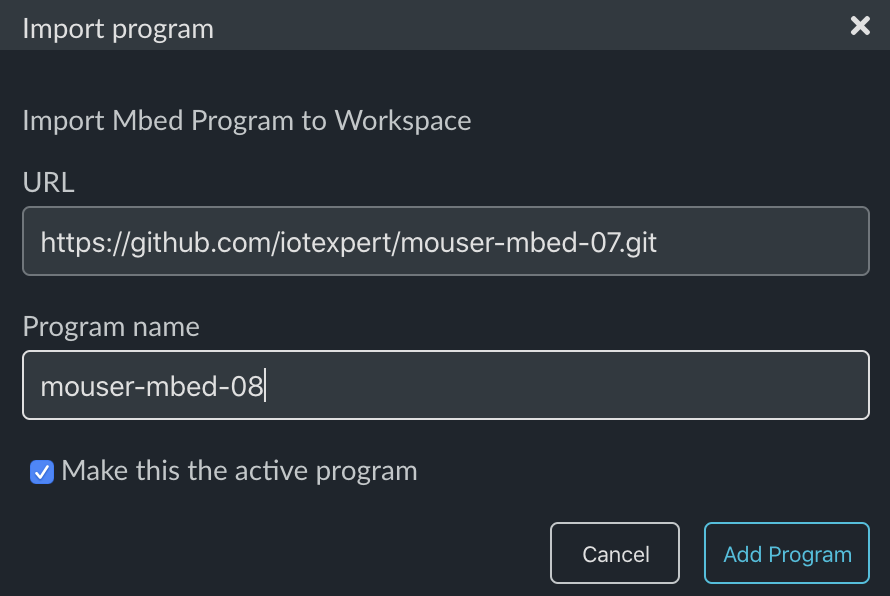
Add the Cypress AWS IoT Middleware
Cypress has built a library which will help you manage connections to AWS. We call it the aws IoT middleware library. Go to the library manager and add it:
- https://github.com/cypresssemiconductorco/aws-iot.git
Use the master branch:
Add the Cypress Connectivity Utilities Library
The AWS IoT library needs to use another library of connectivity functions. Add it:
- https://github.com/cypresssemiconductorco/connectivity-utilities.git
Select the master branch:
Create an AWS Policy, Thing, and Certificate
In order to make a connection to AWS you need to have a policy attached to a certificate. In addition it makes sense to have an AWS “Thing” to represent your system.
Start from the AWS Console and press “Secure->Policies”. Then press “Create”
Give your policy a name. Add all “iot:*” and all resource and “allow”. Then press “Create”:
Once the policy is created you will see it in the catalog:
Create a new Thing by clicking “Manage -> Things”. Then click “Create””
Press “Create a single thing”:
First give your thing a name (in this case mouser) then click “Next”:
Click “Create certificate”
You MUST DOWNLOAD these files now. You will not be given another chance. Press activate:
Which will show the certificate as active. Next press “attach a policy”:
Then pick the Mouser policy:
Now you have your new “Thing”.
Modify the keys to “C” Format
In order to make a connection to AWS you need a valid certificate, the private key that goes with the certificate and the CA certificate of AWS. You just created these files in the previous step.
These keys come looking like this:
-----BEGIN RSA PRIVATE KEY----- MIIEpQIBAAKCAQEAsRLypD2uhZNZgtfPAuX8M3ZFNYcBU/BHulG9CJrETKwmjXOV j1l9U/Tlu3p/hGIFEhrkbqt1SGvPEj8x7U5kyCpJde1JojObW4m2S5T7WuXxd5JQ Y+rqWkyrvwp/1HPFLRB3n3OopGaUcnEZwegsMznUcsi+kZwrUDkqBWFIQbEEKjW8 UzZxqmbmNSCsJQXHCv9OPrKdeisBnkQrpRkTz4uSG3yZI6jG7acx3UZkMcc6DvDo bbz8PoBisZq4JcKthwpQdiV1RdS4aqCc0wq5Jv7DvhifqNcrOK3PT+zkck82jqIC VDd58NkY6Y0URIyqjPCPPIltCbrAB/+u41rrtQIDAQABAoIBAQCQAhvprOxpX+u1 OLP35HjWlYI1xSU0Ub7T7bPx8oRg4sS711uz6JC/nfTUIwzf6iO7lLlgs/q/OkZ+ zXxaRZ47GAEEckWnL5dSu83Q7En7o/RcTVcp25xace5fgTdy3fBm9PSEbjih83cZ F5heFecUhhyceVxa6YpkRQlCtNph6SwFfsY1BmfhHISHh4U7XYityUatdalQfOtL Vf79TRIE/X1aX/H/xXYsqNQPCSGUzNj1BU5aHOM93B+hNg8q1t+Ze5w6Q/HkMBTb 5VPI8fabvaAV6WUrrWtEl8ErcokutYWNRadXH0ieCGiuI2uoK0qqgrBb2MwZwv81 ZtzCm3QlAoGBAOj5zlZtcWN5Mfb9PHonPSwFPE4pud2fZCJjO9btQWb6ELYwp9qW 2aRaoaQm9bA7pTwMaSRQYGC9eKPu8hu+cqPXJZ1gg1GrYP+IfJMChDAM0x7Faks4 B7ZDjfQlRGUJGAkqLQ4/4+k2y75KtZdnri5Z40myh4f0+PuiDDRwPcGjAoGBAMKS 2VuNefMvzqKW7g/drRLzChudUL96r2BWI8nkl7S9UChtKVgdqJcrNSiKXbTv0wLn pWsnlOIxPVJAi1u5we045U+ZQadgIg4UqeIBVfRVKm4ZKrInSCT4uMriGDDJuvQl NXBv9PIX8WCaPWpG2rcsOFsi0mNBcImVHMAhc2LHAoGBAIk6k8Ku5opMWhT9J0Fg mZSzZMk5pMSZXXcv8pBv4gVRKMTYNhb4oixAQlQZqsBq8bJEMS51tb9l+4i8d5nF /WrqkLp5ngBeLV13PMGvSsOu2jCW4jx6PXirpBL6XKYSzDihwjZRheLaJvrosLwF E0E0K0A+y7xWnM5DrmK49nd3AoGAQdp70F24yZMDp8nXdu07F6/EWwZKfxQh6UQe RsWkhtqQF66ikJ0xI0DPdBIolwWYcGJAfVzfKhMqQv1vbTMYrJZWHjOrod+KhyN9 P+3dzp1IiAzig3uCEmlP+fK95z1PljRFuvFZgNqTqnNpl9+1RMulo0rM1CUg1p/u JCTuLZ8CgYEAwUsBplIARj4i+hhyVWC1WOR+I4AqgRAauCwliO9gvToJzjkQsc5q RZxPbXLbm907wy6/7P/tKT20WhZ0b7szZsXchdeX20xSfAa4N0bJTZy3OiS0CYtF 3+Xw1Mlu3Gihr89X9QeSot0tH9m6QZky032aLW/8jbNT3Eb49niqQZA= -----END RSA PRIVATE KEY-----
But you need them to look like this so that they can be read in by the Mbed TLS library.
// Private key const char SSL_CLIENTKEY_PEM[] = \ "-----BEGIN RSA PRIVATE KEY-----\n"\ "MIIEpQIBAAKCAQEAsRLypD2uhZNZgtfPAuX8M3ZFNYcBU/BHulG9CJrETKwmjXOV\n"\ "j1l9U/Tlu3p/hGIFEhrkbqt1SGvPEj8x7U5kyCpJde1JojObW4m2S5T7WuXxd5JQ\n"\ "Y+rqWkyrvwp/1HPFLRB3n3OopGaUcnEZwegsMznUcsi+kZwrUDkqBWFIQbEEKjW8\n"\ "UzZxqmbmNSCsJQXHCv9OPrKdeisBnkQrpRkTz4uSG3yZI6jG7acx3UZkMcc6DvDo\n"\ "bbz8PoBisZq4JcKthwpQdiV1RdS4aqCc0wq5Jv7DvhifqNcrOK3PT+zkck82jqIC\n"\ "VDd58NkY6Y0URIyqjPCPPIltCbrAB/+u41rrtQIDAQABAoIBAQCQAhvprOxpX+u1\n"\ "OLP35HjWlYI1xSU0Ub7T7bPx8oRg4sS711uz6JC/nfTUIwzf6iO7lLlgs/q/OkZ+\n"\ "zXxaRZ47GAEEckWnL5dSu83Q7En7o/RcTVcp25xace5fgTdy3fBm9PSEbjih83cZ\n"\ "F5heFecUhhyceVxa6YpkRQlCtNph6SwFfsY1BmfhHISHh4U7XYityUatdalQfOtL\n"\ "Vf79TRIE/X1aX/H/xXYsqNQPCSGUzNj1BU5aHOM93B+hNg8q1t+Ze5w6Q/HkMBTb\n"\ "5VPI8fabvaAV6WUrrWtEl8ErcokutYWNRadXH0ieCGiuI2uoK0qqgrBb2MwZwv81\n"\ "ZtzCm3QlAoGBAOj5zlZtcWN5Mfb9PHonPSwFPE4pud2fZCJjO9btQWb6ELYwp9qW\n"\ "2aRaoaQm9bA7pTwMaSRQYGC9eKPu8hu+cqPXJZ1gg1GrYP+IfJMChDAM0x7Faks4\n"\ "B7ZDjfQlRGUJGAkqLQ4/4+k2y75KtZdnri5Z40myh4f0+PuiDDRwPcGjAoGBAMKS\n"\ "2VuNefMvzqKW7g/drRLzChudUL96r2BWI8nkl7S9UChtKVgdqJcrNSiKXbTv0wLn\n"\ "pWsnlOIxPVJAi1u5we045U+ZQadgIg4UqeIBVfRVKm4ZKrInSCT4uMriGDDJuvQl\n"\ "NXBv9PIX8WCaPWpG2rcsOFsi0mNBcImVHMAhc2LHAoGBAIk6k8Ku5opMWhT9J0Fg\n"\ "mZSzZMk5pMSZXXcv8pBv4gVRKMTYNhb4oixAQlQZqsBq8bJEMS51tb9l+4i8d5nF\n"\ "/WrqkLp5ngBeLV13PMGvSsOu2jCW4jx6PXirpBL6XKYSzDihwjZRheLaJvrosLwF\n"\ "E0E0K0A+y7xWnM5DrmK49nd3AoGAQdp70F24yZMDp8nXdu07F6/EWwZKfxQh6UQe\n"\ "RsWkhtqQF66ikJ0xI0DPdBIolwWYcGJAfVzfKhMqQv1vbTMYrJZWHjOrod+KhyN9\n"\ "P+3dzp1IiAzig3uCEmlP+fK95z1PljRFuvFZgNqTqnNpl9+1RMulo0rM1CUg1p/u\n"\ "JCTuLZ8CgYEAwUsBplIARj4i+hhyVWC1WOR+I4AqgRAauCwliO9gvToJzjkQsc5q\n"\ "RZxPbXLbm907wy6/7P/tKT20WhZ0b7szZsXchdeX20xSfAa4N0bJTZy3OiS0CYtF\n"\ "3+Xw1Mlu3Gihr89X9QeSot0tH9m6QZky032aLW/8jbNT3Eb49niqQZA=\n"\ "-----END RSA PRIVATE KEY-----";
There are a number of ways to change the format including manual editing.
For this lesson I put the keys into a file called “aws_config.h” There are bunches of bad stories out there about people finding encryption keys on the internet (like these). I am going to disable the keys as soon as this workshop is over, but you should be careful with yours.
Here is my aws_config.h
/* Change device certificate and device private key based on your account */ // certificate const char SSL_CLIENTCERT_PEM[] = \ "-----BEGIN CERTIFICATE-----\n\ MIIDWjCCAkKgAwIBAgIVAJ4u/gU7NxWiV2NwBRTFJk/mPPkvMA0GCSqGSIb3DQEB\n\ CwUAME0xSzBJBgNVBAsMQkFtYXpvbiBXZWIgU2VydmljZXMgTz1BbWF6b24uY29t\n\ IEluYy4gTD1TZWF0dGxlIFNUPVdhc2hpbmd0b24gQz1VUzAeFw0xOTA3MDMxMTM5\n\ MTZaFw00OTEyMzEyMzU5NTlaMB4xHDAaBgNVBAMME0FXUyBJb1QgQ2VydGlmaWNh\n\ dGUwggEiMA0GCSqGSIb3DQEBAQUAA4IBDwAwggEKAoIBAQCxEvKkPa6Fk1mC188C\n\ 5fwzdkU1hwFT8Ee6Ub0ImsRMrCaNc5WPWX1T9OW7en+EYgUSGuRuq3VIa88SPzHt\n\ TmTIKkl17UmiM5tbibZLlPta5fF3klBj6upaTKu/Cn/Uc8UtEHefc6ikZpRycRnB\n\ 6CwzOdRyyL6RnCtQOSoFYUhBsQQqNbxTNnGqZuY1IKwlBccK/04+sp16KwGeRCul\n\ GRPPi5IbfJkjqMbtpzHdRmQxxzoO8OhtvPw+gGKxmrglwq2HClB2JXVF1LhqoJzT\n\ Crkm/sO+GJ+o1ys4rc9P7ORyTzaOogJUN3nw2RjpjRREjKqM8I88iW0JusAH/67j\n\ Wuu1AgMBAAGjYDBeMB8GA1UdIwQYMBaAFIJyQZprW3SRNIXe+X22/uZ6sdO9MB0G\n\ A1UdDgQWBBT+al32O2+dH5qoClpyEWRRoMk6oTAMBgNVHRMBAf8EAjAAMA4GA1Ud\n\ DwEB/wQEAwIHgDANBgkqhkiG9w0BAQsFAAOCAQEAoRg4QETRC1rMKJytxthLuGDj\n\ WtB9Zow3TONnq1HZ4yMUQFQeflYCZ96khBuWu91cj2iZengikEJkl+6HGCL+9ZKA\n\ ybWPRyQNvJQ13ju/iQyl89ZIbYh5UGmGrk6G8ryyvPezVP8AxpB5usz9YxTTz/m6\n\ eQMs38zUK5Dv4297NBJ6Xxwoqb8ivJkHEmnkDxKeErd4Qb7Q9ukWYlo5ksjqib8F\n\ NYTv8fhcx4S3UFhbNB+z/iaGtJXk7WIzgGpSX/CiRtGcV776c94LdgIfIZ9DpLgi\n\ GWSrCjsCr6yNtc708vwC+0jKoBcrk3HdVII2N4ciNuzJaoGyuHi+YuiXnO8kbg==\n\ -----END CERTIFICATE-----"; // Private key const char SSL_CLIENTKEY_PEM[] = \ "-----BEGIN RSA PRIVATE KEY-----\n"\ "MIIEpQIBAAKCAQEAsRLypD2uhZNZgtfPAuX8M3ZFNYcBU/BHulG9CJrETKwmjXOV\n"\ "j1l9U/Tlu3p/hGIFEhrkbqt1SGvPEj8x7U5kyCpJde1JojObW4m2S5T7WuXxd5JQ\n"\ "Y+rqWkyrvwp/1HPFLRB3n3OopGaUcnEZwegsMznUcsi+kZwrUDkqBWFIQbEEKjW8\n"\ "UzZxqmbmNSCsJQXHCv9OPrKdeisBnkQrpRkTz4uSG3yZI6jG7acx3UZkMcc6DvDo\n"\ "bbz8PoBisZq4JcKthwpQdiV1RdS4aqCc0wq5Jv7DvhifqNcrOK3PT+zkck82jqIC\n"\ "VDd58NkY6Y0URIyqjPCPPIltCbrAB/+u41rrtQIDAQABAoIBAQCQAhvprOxpX+u1\n"\ "OLP35HjWlYI1xSU0Ub7T7bPx8oRg4sS711uz6JC/nfTUIwzf6iO7lLlgs/q/OkZ+\n"\ "zXxaRZ47GAEEckWnL5dSu83Q7En7o/RcTVcp25xace5fgTdy3fBm9PSEbjih83cZ\n"\ "F5heFecUhhyceVxa6YpkRQlCtNph6SwFfsY1BmfhHISHh4U7XYityUatdalQfOtL\n"\ "Vf79TRIE/X1aX/H/xXYsqNQPCSGUzNj1BU5aHOM93B+hNg8q1t+Ze5w6Q/HkMBTb\n"\ "5VPI8fabvaAV6WUrrWtEl8ErcokutYWNRadXH0ieCGiuI2uoK0qqgrBb2MwZwv81\n"\ "ZtzCm3QlAoGBAOj5zlZtcWN5Mfb9PHonPSwFPE4pud2fZCJjO9btQWb6ELYwp9qW\n"\ "2aRaoaQm9bA7pTwMaSRQYGC9eKPu8hu+cqPXJZ1gg1GrYP+IfJMChDAM0x7Faks4\n"\ "B7ZDjfQlRGUJGAkqLQ4/4+k2y75KtZdnri5Z40myh4f0+PuiDDRwPcGjAoGBAMKS\n"\ "2VuNefMvzqKW7g/drRLzChudUL96r2BWI8nkl7S9UChtKVgdqJcrNSiKXbTv0wLn\n"\ "pWsnlOIxPVJAi1u5we045U+ZQadgIg4UqeIBVfRVKm4ZKrInSCT4uMriGDDJuvQl\n"\ "NXBv9PIX8WCaPWpG2rcsOFsi0mNBcImVHMAhc2LHAoGBAIk6k8Ku5opMWhT9J0Fg\n"\ "mZSzZMk5pMSZXXcv8pBv4gVRKMTYNhb4oixAQlQZqsBq8bJEMS51tb9l+4i8d5nF\n"\ "/WrqkLp5ngBeLV13PMGvSsOu2jCW4jx6PXirpBL6XKYSzDihwjZRheLaJvrosLwF\n"\ "E0E0K0A+y7xWnM5DrmK49nd3AoGAQdp70F24yZMDp8nXdu07F6/EWwZKfxQh6UQe\n"\ "RsWkhtqQF66ikJ0xI0DPdBIolwWYcGJAfVzfKhMqQv1vbTMYrJZWHjOrod+KhyN9\n"\ "P+3dzp1IiAzig3uCEmlP+fK95z1PljRFuvFZgNqTqnNpl9+1RMulo0rM1CUg1p/u\n"\ "JCTuLZ8CgYEAwUsBplIARj4i+hhyVWC1WOR+I4AqgRAauCwliO9gvToJzjkQsc5q\n"\ "RZxPbXLbm907wy6/7P/tKT20WhZ0b7szZsXchdeX20xSfAa4N0bJTZy3OiS0CYtF\n"\ "3+Xw1Mlu3Gihr89X9QeSot0tH9m6QZky032aLW/8jbNT3Eb49niqQZA=\n"\ "-----END RSA PRIVATE KEY-----"; const char SSL_CA_PEM[] = \ "-----BEGIN CERTIFICATE-----\n"\ "MIIDQTCCAimgAwIBAgITBmyfz5m/jAo54vB4ikPmljZbyjANBgkqhkiG9w0BAQsF\n"\ "ADA5MQswCQYDVQQGEwJVUzEPMA0GA1UEChMGQW1hem9uMRkwFwYDVQQDExBBbWF6\n"\ "b24gUm9vdCBDQSAxMB4XDTE1MDUyNjAwMDAwMFoXDTM4MDExNzAwMDAwMFowOTEL\n"\ "MAkGA1UEBhMCVVMxDzANBgNVBAoTBkFtYXpvbjEZMBcGA1UEAxMQQW1hem9uIFJv\n"\ "b3QgQ0EgMTCCASIwDQYJKoZIhvcNAQEBBQADggEPADCCAQoCggEBALJ4gHHKeNXj\n"\ "ca9HgFB0fW7Y14h29Jlo91ghYPl0hAEvrAIthtOgQ3pOsqTQNroBvo3bSMgHFzZM\n"\ "9O6II8c+6zf1tRn4SWiw3te5djgdYZ6k/oI2peVKVuRF4fn9tBb6dNqcmzU5L/qw\n"\ "IFAGbHrQgLKm+a/sRxmPUDgH3KKHOVj4utWp+UhnMJbulHheb4mjUcAwhmahRWa6\n"\ "VOujw5H5SNz/0egwLX0tdHA114gk957EWW67c4cX8jJGKLhD+rcdqsq08p8kDi1L\n"\ "93FcXmn/6pUCyziKrlA4b9v7LWIbxcceVOF34GfID5yHI9Y/QCB/IIDEgEw+OyQm\n"\ "jgSubJrIqg0CAwEAAaNCMEAwDwYDVR0TAQH/BAUwAwEB/zAOBgNVHQ8BAf8EBAMC\n"\ "AYYwHQYDVR0OBBYEFIQYzIU07LwMlJQuCFmcx7IQTgoIMA0GCSqGSIb3DQEBCwUA\n"\ "A4IBAQCY8jdaQZChGsV2USggNiMOruYou6r4lK5IpDB/G/wkjUu0yKGX9rbxenDI\n"\ "U5PMCCjjmCXPI6T53iHTfIUJrU6adTrCC2qJeHZERxhlbI1Bjjt/msv0tadQ1wUs\n"\ "N+gDS63pYaACbvXy8MWy7Vu33PqUXHeeE6V/Uq2V8viTO96LXFvKWlJbYK8U90vv\n"\ "o/ufQJVtMVT8QtPHRh8jrdkPSHCa2XV4cdFyQzR1bldZwgJcJmApzyMZFo6IQ6XU\n"\ "5MsI+yMRQ+hDKXJioaldXgjUkK642M4UwtBV8ob2xJNDd2ZhwLnoQdeXeGADbkpy\n"\ "rqXRfboQnoZsG4q5WTP468SQvvG5\n"\ "-----END CERTIFICATE-----";
Create and Code awsThread.h
Now create a new header file for the awsThread:
This file just contains the thread function prototype.
#ifndef AWS_THREAD_H #define AWS_THREAD_H void awsThread(void); #endif
Create and Code awsThread.cpp
Now create the awsThread.cpp file for the aws implementation:
The basis for this code is again taken from a code example that is provided by Cypress on GitHub. You can find that code example here:
github.com/cypresssemiconductorco/aws-iot
The awsThread starts with my evil extern.
Then I declare a function which is called when a message comes in from the MQTT broker. I ASSUME that the message is an ASCII message formatted as %2.1f and I use sscanf to convert it to a float. Many bugs have been made with this EXACT scheme. It is expedient way to show people but it is a horrible habit. I then send it to the temperature controller.
The main awsThread starts by initializing the AWS Client library, then connecting to AWS. Lastly it subscribes to the setPoint topic. Then it goes into an infinite loop calling the yield function which is responsible to listening to the aws MQTT socket and doing something with the data… specifically calling the callback.
#include "mbed.h" #include "aws_client.h" #include "aws_config.h" #include "temperatureThread.h" #define AWSIOT_ENDPOINT_ADDRESS "a1c0l0bpd6pon3-ats.iot.us-east-2.amazonaws.com" extern WiFiInterface *wifi; static void messageArrived(aws_iot_message_t& md) { float setPoint; aws_message_t &message = md.message; sscanf((char*)message.payload,"%f",&setPoint); tempSendUpdateSetpointF(setPoint); } void awsThread(void) { AWSIoTClient client; AWSIoTEndpoint* ep = NULL; /* Initialize AWS Client library */ AWSIoTClient AWSClient(wifi, "thermostat" , SSL_CLIENTKEY_PEM, strlen(SSL_CLIENTKEY_PEM), SSL_CLIENTCERT_PEM, strlen(SSL_CLIENTCERT_PEM)); aws_connect_params conn_params = { 0,0,NULL,NULL,NULL,NULL,NULL }; ep = AWSClient.create_endpoint(AWS_TRANSPORT_MQTT_NATIVE, AWSIOT_ENDPOINT_ADDRESS, 8883, SSL_CA_PEM, strlen(SSL_CA_PEM)); /* set MQTT connection parameters */ conn_params.username = NULL; conn_params.password = NULL; conn_params.keep_alive = 60; conn_params.peer_cn = (uint8_t*) AWSIOT_ENDPOINT_ADDRESS; conn_params.client_id = (uint8_t*)"thermostat"; /* connect to an AWS endpoint */ AWSClient.connect (ep, conn_params); AWSClient.subscribe(ep, "setPoint", AWS_QOS_ATMOST_ONCE, messageArrived); while(1) { AWSClient.yield(1000); } }
Update main.cpp
The last step is to start up the awsThread in main:
#include "mbed.h" #include "blinkThread.h" #include "displayThread.h" #include "temperatureThread.h" #include "capsenseThread.h" #include "ntpThread.h" #include "awsThread.h" Thread blinkThreadHandle; Thread displayThreadHandle; Thread temperatureThreadHandle; Thread capsenseThreadHandle; Thread ntpThreadHandle; Thread awsThreadHandle; WiFiInterface *wifi; int main() { printf("Started System\n"); int ret; wifi = WiFiInterface::get_default_instance(); do { ret = wifi->connect("CYFI_IOT_EXT", "cypresswicedwifi101", NSAPI_SECURITY_WPA_WPA2); if (ret != 0) { ThisThread::sleep_for(2000); // If for some reason it doesnt work wait 2s and try again } } while(ret !=0); ntpThreadHandle.start(ntpThread); blinkThreadHandle.start(blinkThread); displayThreadHandle.start(displayThread); temperatureThreadHandle.start(temperatureThread); capsenseThreadHandle.start(capsenseThread); awsThreadHandle.start(awsThread); }
Build, Program and Test
When you build and program the development kit. You will be able to publish messages to the “setPoint” topic which will then change the setPoint on your system. Remember that my parser is really not safe so just send %2.1f.
In the case below you can see that I publish 51.1:
Which changes the setPoint on my board.
2 Comments
It looks like the Cypress Connectivity Utilities and Cypress AWS IoT Middleware Library has been updated and there’s error when I follow this code. Is there an updated version of the code or any tips on how to code using the updated Library?
Hopefully at this point this is fixed… sorry for the way slow response.
Alan